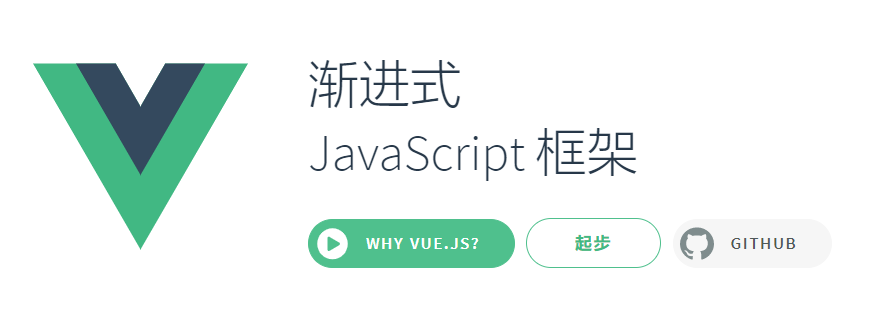
vue2笔记下
重新整理了一下vue2下
[TOC]
vue2
scoped 样式
查看某个包的每个版本
作用: 让样式在局部生效,防止冲突
写法:
<style scoped><style>
TodoList 案例_静态
组件名建议多个单词小驼峰名字
id:使用 uuid
父传子: :属性名=”属性值”
data(){
return{
属性值:[]
}
}
子页面接收:
props:['属性名']
儿子传父亲:
this.$emit()
或者 this. props 中接收的属性名
reduce:Es6 条件统计 常用于求和
全选操作:
父元素:
子元素:
v-mode 写法:
//清除全部:
父元素:
子元素:
总结:
组件话编码流程
- 拆分静态组件:组件要按照功能点拆分,命名不要与 html 元素冲突
- 实现动态组件:考虑好数据的存放位置,数据是一个组件在用,还是一些组件再用
(1).一个组件在用:放在组件自身即可。
(2).一些组件再用:放在他们公共的父组件上(状态提升)
(3).实现交互:从绑定事件开始。
props 适用于:
- 父组件 ==> 子组件 通信
- 子组件 ==> 父组件 通信(要求父给子一个函数)
使用 v-model 时要切记:v-model 绑定的值不能是 props 传过来的值,因为 props 是不可以修改的!
props 传过来的若是对象类型的值,修改对象中的属性时 Vue 不会报错,但不推荐这样做。
浏览器本地存储
localStorage
localStorage.setItem('key','value') //存储数据
存储进去的都是字符串:
传递进去的是一个对象得转换成json格式 JSON.stringify(需要被转换的函数)
函数.Join('')
------------------------------------
localStorage.getItem('key') //读取数据
读取json格式的方法 JSON.parse(函数)
------------------------------------
localStorage.removeItem('key') //删除数据
------------------------------------
localStorage.clear() //清空
sessionStorage
总结:
localStorage和sessionStorage 统称为 webStorage
- 存储内容大小一般支持 5mb 左右
- 浏览器端通过 Window.sessionStorage 和 Window.localStorage 属性来实现本地存储机制。
- 相关 API:
- xxxxStorage.setItem(‘key’,’value’) //该方法接收一个键和值作为参数,会把键值添加到存储中,如果键名存在,则更新其对应的值
- xxxxStorage.getItem(‘person’) //该方法接受一个键名作为参数,返回键名对应的值
- xxxxStorage.removeItem(‘key’) //该方法接收一个键名作为参数,并把该键名从存储中删除。
- xxxxStorage.clear() //该方法会清空存储中的所有数据。
- 备注:
- sessionStorage 存储的内容会随着浏览器窗口关闭而消失。
- localStorage 存储的内容,需要手动清除才会消失。
- xxxxStorage.getItem(‘xxx’) 如果 xxxx 对应的 value 获取不到,那么 getItem 的返回值是 null
- JSON.parse(null)的结果依然是 null
自定义事件——绑定
组件自定义事件——解绑
this.$off('事件名') //解绑一个自定义事件
this.$off(['事件名','事件名']) //解绑多个自定义事件
this.$off() //解绑所有的自定义事件
补充:
this.$destroy() 销毁了当前的组件实例,销毁后所有组件实例的自定义事件全都不奏效
组件自定义事件_总结
在组件身上写 @click 是认为自定义属性 如果我们想要触发 需要写 .native 修饰符
一种组件间通信方式,适用于: 子组件===》父组件
使用场景:A 是父组件,B 是子组件,B 想给 A 传数据,那么就要 A 中给 B 绑定自定义事件(事件的回调在 A 中)
绑定自定义事件:
- 第一种方式,在父组件中 <Demo @abc=”test” /> 或者
- 第二种方式:在父组件中:
<Demo @abc="test" /> ... mounted(){ this.$refs.xxx.$on('abc',this.test) }
- 若想让自定义事件只能触发一次,可以使用 once 修饰符,或者$once 方法
- 第一种方式,在父组件中 <Demo @abc=”test” /> 或者
触发自定义事件: this.$emit(‘abc’,数据)
解绑自定义事件:this.$off(‘abc’)
组件上也可以绑定原生 Dom 事件,需要使用 native 修饰符。
注意:通过 this.$refs.xxx.$on(‘abc’,回调)绑定自定义事件时,回调要么配置 methods 中,要么用箭头函数,否则 this 指向会出问题!
全局事件总线 (任意组件间通信)
全局事件总线(GlobalEventBus)
一种组件间通信的方式,适用于任意组件间通信
安装全局事件总线:
new Vue({ ...... beforeCreate(){ Vue.prototype.$bus = this //安装全局事件总线,$bus就是当前应用的vm }, ...... })
使用事件总线:
- 接收数据:A 组件想接收数据,则 A 组件中给$bus 绑定自定义事件,事件的回调留在 A 组件自身
methods(){ demo(data){.......} } ...... mounted(){ this.$bus.$on('事件名',this.demo) }
- 提供数据:this.$bus.$emit(‘事件名’,数据)
最好在 beforeDestroy 钩子中,用$off 去解绑当前组件所用到的事件。
消息订阅与发布——pubsub(github 上的库)下载:npm i pubsub-js
//接收
mounted(){
this.pubId = pubsub.subscribe('事件名',(消息名,参数)=>{
console.log(消息名,参数)
})
}
//取消
beforeDestroy(){
pubsub.unsubscribe(this.pubId) //类似取消定时器一样
}
---------------------------------------------------------------------
//发布
pubsub.publish('发布的消息',参数)
总结
- 一种组件间通信的方式,适用于任意组件间通信
- 使用步骤
- 安装 pubsub:npm i pubsub-js
- 引入: import pubsub from ‘pubsub-js’ //pubsub 是一个对象
- 接收数据:A 组件想接收数据,则在 A 组件中订阅消息,订阅的回调留在 A 组件自身
methods(){
data(data){.......}
}
......
mounted(){
this.pid = pubsub.subscribe('xxx',this.demo) //订阅消息
}
- 提供数据:pubsub.publish(‘xxx’,数据)
- 最好在 beforeDestroy 钩子中,用 pubsub.unsubscribe(this.pid) 去取消 这个 this.pid 是类似定时器一样去取消
$nextTick
- 语法:this.$nextTick(回调函数)
- 作用:在下一次 DOM 更新结束后执行其指定的回调
- 什么时候用:当改变数据后,要基于更新后的新 DOM 进行某些操作时,要在 nextTick 所指定的回调函数中执行。
动画效果
过渡
动画
多个元素过渡
集成第三方动画
动画库:animate.css
总结
作用:在插入、更新或溢出 DOM 元素时,在核实的时候给元素添加样式类名
图示:
写法:
- 准备好样式:
- 元素进入的样式
- v-enter:进入的起点
- v-enter-active:进入过程中
- v-enter-to:进入的重点
- 元素离开的样式
- v-leave:离开的起点
- v-leave-active:离开的过程中
- v-leave-to:离开的终点
- 元素进入的样式
- 使用
包裹要过渡的元素,并配置 name 属性:
<transition name="hello"> <h1 v-show="isShow">你好啊!<h1> </transition>
- 备注:若有多个元素需要过渡,则需要使用:
且每个元素都要指定 key 值
- 准备好样式:
配置代理
方式一:
脚手架里面的:代理服务器:devServer.proxy
在 vue.config.js 中添加如下配置:
devServer:{
proxy:'http://localhost:5000'
}
说明:
- 优点:配置简单,请求资源时直接发给前端(8080)即可
- 缺点:不能配置多个代理,不能灵活的控制请求是否走代理
- 工作方式:若按照上述配置代理,当请求了前端不存在的资源时,那么该请求会转发给服务器(有限匹配前端资源)
方式二:
编写 vue.config.js 配置具体代理规则:
module.exports = {
devServer: {
proxy: {
'/api': { //匹配所有以 '/api'开头的请求路径
target: '<url>', //代理目标的基础路径
ws: true, //用于支持websocket
changeOrigin: true, //用于控制请求头中的host值
pathRewrite: {'^/api':''} //用正则去匹配所有的/api 替换为''
},
'/foo': { //配置第二个请求路径
target: '<other_url>'
}
}
}
}
说明:
- 优点: 可以配置多个代理,且可以灵活的控制请求是否走代理。
- 缺点:配置略微繁琐,请求资源时必须加前缀
vue-resource ajax 组件库
slot 插槽
- 作用:让父组件可以想子组件指定位置插入 html 解构,也是一种组件间通信方式,适用于父组件 ===> 子组件
- 分类:默认插槽,具名插槽,作用于插槽
- 使用方式:
- 默认插槽:
父组件中:
<AbcGory>
<div>html解构</div>
</AbcGory>
子组件中:
<template>
<div>
<!-- 定义插槽 -->
<slot>....插槽默认内容....</slot>
</div>
</template>
- 具名插槽:
父组件中:
<AbcGory>
<template slot="center">
<div>html解构</div>
</template>
<template v-slot:footer>
<div>htm2解构</div>
</template>
</AbcGory>
子组件中:
<template>
<div>
<!-- 定义插槽 -->
<slot name="center">插槽默认内容....</slot>
<slot name="footer">插槽默认内容....</slot>
</div>
</template>
- 作用于插槽:
- 理解: 数据在组件的自身,但根据数据生成的结构需要组件的使用者来决定。(games 在 AbcGory 组件中,但使用数据所遍历出来的结构由 App 组件来决定)
- 具体编码:
父组件中:
<AbcGory>
<template slot="{ games }">
<!-- 生成的是ul列表 -->
<ul>
<li v-for="item in games" :key="item">{{ item }}</li>
</ul>
</template>
</AbcGory>
<AbcGory>
<!-- 说一下这个{ games } 这是解构-->
<template slot-scope="{ games }">
<!-- 生成的是h4标题 -->
<h4 v-for="(item.index) in games" :key="index">{{ item }}</h4>
</template>
</AbcGory>
子组件中:
<template>
<div>
<slot :games="games"></slot>
</div>
</template>
<script>
export default {
name: 'AbcGory',
props:['title'],
<!-- 数据在子组件自身 -->
data(){
return{
games:['11231','2131sa','2131sd']
}
}
}
</script>
vuex 状态管理包
- 理解 vuex
- vuex 是什么
- 概念:专门在 vue 中是西安集中式状态(数据)管理的一个 vue 插件,对 vue 应用中多个组件的共享状态进行集中式管理(读/写),也是一种组件间通信的方式,且适用于任意组件间通信
- Github 地址:https://github.com/vuejs/vuex
- 什么时候使用 vuex
- 多个组件依赖于同一个状态
- 来自不同组件的行为需要变更同一状态
- vuex 是什么
多组件共享数据 —– 全局事件总线实现
多组件共享数据 —- vuex 实现
vuex
vuex 概念 2
- 搭建 vuex 环境
import Vue from "vue";
import Vuex from "vuex";
Vue.use(Vuex);
//创建并导出store
export default new Vuex.Store({
//用于存储数据
state: {},
getters: {},
// 用于操作数据
mutations: {},
//用于响应组件中的动作
actions: {},
modules: {},
});
- 在 main.js 中创建 vm 时传入 store 配置项
import Vue from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'
Vue.config.productionTip = false
new Vue({
router,
store,
render: h => h(App)
}).$mount('#app')
- 使用场景
<template>
<div>
<h1>当前求和为:{{ $store.state.sum }}</h1>
<select v-model.number="num">
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<button @click="increment">+</button>
<button @click="decrement">-</button>
<button @click="incrementOdd">当前求和为奇数再加</button>
<button @click="incrementWait">等一等在加</button>
</div>
</template>
<script>
export default {
name: 'Count',
data() {
return {
num: 1, //用户选择的数字
}
},
components: {},
methods: {
increment() {
// 给store身上去创建一个方法 jia
this.$store.commit('JIA', this.num)
},
decrement() {
this.$store.commit('JE', this.num)
},
incrementOdd() {
this.$store.dispatch('he', this.num)
},
incrementWait() {
this.$store.dispatch('dhe', this.num)
},
},
// computed() {},
}
</script>
<style scoped>
button {
margin-right: 5px;
}
</style>
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
//创建并导出store
export default new Vuex.Store({
//用于存储数据
state: {
sum: 0, //求和的数据
},
getters: {},
// 用于操作数据
mutations: {
// 下边的两个参数分别是 state 和 value
JIA(state, value) {
// console.log('JIA被使用了', state, value);
//那么我们就进行加工求和
state.sum += value
},
JE(state, value) {
state.sum -= value
},
},
//用于响应组件中的动作
actions: {
// // 求和 没啥意义所以注释 直对话commit
// jia(context, value) {
// // console.log("jia被使用了", context, value);
// context.commit('JIA', value)
// },
// // 求减
// je(context, value) {
// context.commit('JE', value)
// },
// 两个参数分别是 context 和value// 执行的是迷你版的store
// 求差
he(context, value) {
if (context.state.sum % 2) {
context.commit('JIA', value)
}
},
// 等待求和
dhe(context, value) {
setTimeout(() => {
context.commit('JIA', value)
}, 500)
},
},
modules: {},
})
- 组件中读取 vuex 中的数据:$store.state.sum
- 组件中修改 vuex 中的数据:$store.dispatch(‘action中的方法名’,数据)或$store.commit(‘mutations 中的方法名’,数据)
备注:若没有网络请求或其他业务逻辑,组件中也可以越过 actions,即不写 dispatch,直接编写 commit
store 配置项:
getters
- 概念:当 state 中的数据需要经过加工后再使用时,可以使用 getters 加工
- 在 store.js 中追加 getters 配置
// 用于讲state中的数据进行加工 收到的参数是state
getters: {
BigSum(state) {
// 需要返回一个结果
return state.sum * 10
},
},
- 组件中读取数据:$store.getters.BigSum
四个 map 方法的使用
- mapState 方法:用于帮助我们映射 state 中的数据为计算属性
computed:{
//借助mapState生成计算属性:sum、school、subject(对象方式)
...mapState({sum:'sum',school:'school',subject:'subject'}),
//借助mapState生成计算属性:sum、school、subject(数组写法),
...mapState(['sum','school','subject'])
}
- mapGetters 方法:用于帮助我们映射 getters 中的数据为计算属性
computed:{
// 借助mapGetters生成计算属性,bigSum(对象写法)
...mapGetters({bigSum:'bigSum'})
// 借助mapGetters生成计算属性,bigSum(数组写法)
...mapGetters(['bigSum'])
}
- mapActions 方法:用于帮助我们生成与 actions 对话的方法,即包含$store.dispatch(xxx)的函数
methods:{
// 靠mapActions生成,incrementOdd、incrementWait(对象形式)
...mapActions({incrementOdd:'jiaOdd',incrementWait:'jiaWait'})
// 靠mapActions生成,incrementOdd、incrementWait(数组形式)
...mapActions(['jiaOdd','jiaWait'])
}
- mapMutations 方法:用于帮助我们生成与 mutations 对话的方法,即:包含$store.commit(xxx)的函数
methods:{
// 靠 mapMutations 生成,incrementOdd、incrementWait(对象形式)
…mapMutations({incrementOdd:’jiaOdd’,incrementWait:’jiaWait’})
// 靠 mapMutations 生成,incrementOdd、incrementWait(数组形式)
…mapMutations([‘jiaOdd’,’jiaWait’])
}
备注:mapActions 与 mapMutations 使用时,若需要传递参数需要:在模板中绑定事件时传递好参数,否则参数是事件对象
模块化+命名空间
- 目的:让代码更好维护,让多种数据分类更加明确
- 修改文件
- 开始命名空间后,组件中读取 state 数据:
<!-- 方式一:自己直接读取 -->
this.$store.personAbout.list
<!-- 方式二:借助mapState读取 -->
...mapState['countAbout',['sum','school','subject']]
- 开启命名空间后,组件中去读 getters 数据:
<!-- 方式一:自己直接读取 -->
this.$store.getters['personAbout/fis']
<!-- 方式二:借助mapState读取 -->
...mapGetters['countAbout',['bigSum']]
- 开启命名空间后,组件中调用 dispatch
<!-- 方式一:自己直接读取 -->
this.$store.dispatch('personAbout/add',person)
<!-- 方式二:借助mapState读取 -->
...mapActions['countAbout',[{increment:'jiaOdd',incrementWait:'jiaWait'}]]
- 开启命名空间后,组件中调用 commit
<!-- 方式一:自己直接读取 -->
this.$store.commit('personAbout/Add_PERSON',person)
<!-- 方式二:借助mapState读取 -->
...mapState['countAbout',[increment:'JIA',decrement:'JIAn']]
路由
vue-router 的理解
vue 的一个插件库,准们用来实现 SPA 应用
对 SPA 用用的理解
- 但也没 Web 应用(Single page web application,SPA)
- 整个应用只有一个完成的页面
- 点击页面中导航链接不会刷新页面,只会做页面的局部更新
- 数据需要通过 ajax 请求获取
路由的理解
- 什么是路由?
- 一个路由就是一组映射关系(key - value)
- key 为路径,value 可能是 function 或 component
- 路由分类
- 后端路由:
- 理解:value 是 function,用于处理客户端提交的请求。
- 工作过程:服务器收到一个请求时,根据请求路径找到匹配的函数来处理请求,返回响应数据
- 前端路由:
- 理解:value 是 component,用于展示页面内容
- 工作过程:当浏览器的路径改变时,对应的组件就会显示
- 后端路由:
基本路由
- 安装 vue-router,命令:npm i vue-router
- 应用插件 Vue.use(VueRouter)
- 编写 router 配置项
import Vue from 'vue'
// 引入VueRouter
import VueRouter from 'vue-router'
//挂载VueRouter
Vue.use(VueRouter)
// 创建router实例对象,去管理一组一组的路由规则
const routes = [
{
path: '/about',
name: 'about',
component: () => import('@/views/about.vue'),
},
{
path: '/home',
name: 'home',
component: () => import('@/views/home.vue'),
},
]
const router = new VueRouter({
routes,
})
//暴露Router
export default router
实现切换(active-class 可配置高亮样式)
active-class="active"
指定展示位置
<router-view></router-view>
几个注意点
- 路由组件通常存放在 pages 文件夹,一般组件通常存放在 components 文件夹
- 通过切换,“隐藏”了的路由组件,默认是被销毁掉的,需要的时候再去挂载
- 每个组件都有自己的$route 属性,里面存储着自己的路由信息
- 整个应用只有一个 router,可以通过组件的$router 属性获取到
嵌套(多级)路由
- 配置路由规则,使用 children 配置项:
import Vue from 'vue'
// 引入VueRouter
import VueRouter from 'vue-router'
//挂载VueRouter
Vue.use(VueRouter)
// 创建router实例对象,去管理一组一组的路由规则
const routes = [
{
path: '/about',
name: 'about',
component: () => import('@/components/about.vue'),
children: [],
},
{
path: '/home',
name: 'home',
component: () => import('@/components/home.vue'),
children: [
{
path: 'ness',
component: () => import('@/pages/neww.vue'),
},
{
path: 'massage',
component: () => import('@/pages/message.vue'),
},
],
},
]
const router = new VueRouter({
routes,
})
//暴露Router
export default router
跳转(要写完整路径):
<li> <router-link class="list-group-item" active-class="active" to="/home/ness" >News</router-link > </li> <li> <router-link class="list-group-item" active-class="active" to="/home/massage" >Message</router-link > </li>
路由传参
- 路由的 to query 参数
- 传递参数:
- to 的字符串写法
<router-link :to="`/home/massage/detile?id=${item.id}&title=${item.title}`">{{ item.title }}</router-link>
- to 的对象写法
<router-link
:to="{
path: '/home/massage/detile',
query: {
id: item.id,
title: item.title,
},
}"
>{{ item.title }}</router-link
1. 接收参数:
{{ $route.query.id }}
命名路由
- 作用:可以简化路由的跳转
- 如何使用
- 给路由命名:
// 创建router实例对象,去管理一组一组的路由规则
const routes = [
{
path: '/about',
//命名路由
name: 'about',
component: () => import('@/components/about.vue'),
children: [],
},
{
path: '/home',
name: 'home',
component: () => import('@/components/home.vue'),
children: [
{
path: 'ness',
component: () => import('@/pages/neww.vue'),
},
{
path: 'massage',
component: () => import('@/pages/message.vue'),
children: [
{
//命名路由
name: 'xiangqing',
path: 'detile',
component: () => import('@/components/Detile.vue'),
},
],
},
],
},
]
- 简化跳转:
<!-- 简化前,需要写完整的路径 -->
<router-link class="list-group-item" active-class="active" to="/home/massage">Message<router-link>
<!-- 简化后,直接配合名字进行跳转 -->
<router-link class="list-group-item" active-class="active" :to="{name:'xiaoxi'}" >Message<router-link>
<!-- 简化前 -->
<router-link
:to="{
path: '/home/massage/detile',
query: {
id: item.id,
title: item.title,
},
}"
>{{ item.title }}</router-link
>
<!-- 简化后 配合传递参数 -->
<router-link
:to="{
name: 'xiangqing',
query: {
id: item.id,
title: item.title,
},
}"
>{{ item.title }}</router-link
>
路由的 params 参数
- 配置路由,声明接收 params 参数
{
path: '/home',
name: 'home',
component: () => import('@/components/home.vue'),
children: [
{
path: 'ness',
component: () => import('@/pages/neww.vue'),
},
{
//命名路由
name: 'xiaoxi',
path: 'massage',
component: () => import('@/pages/message.vue'),
children: [
{
//命名路由
name: 'xiangqing',
path: 'detile/:id/:title',
component: () => import('@/components/Detile.vue'),
},
],
},
],
},
- 传递参数
<!-- 跳转路由并携带params参数,to的字符串写法 -->
<router-link :to="`/home/massage/detile/${item.id}/${item.title}`">{{ item.title }}</router-link>
<!-- 跳转路由并携带params参数,to的字符串写法 -->
<router-link :to="{name: 'xiangqing',params: {id: item.id,title: item.title,},}">{{ item.title }}</router-link>
特别注意:路由携带params参数时,若使用to的对象写法,则不能使用path配置项,必须使用name配置!
- 接收参数
{{ $route.params.id }}
{{ $route.params.title }}
路由的 props 配置
作用:让路由组件更方便的收到参数
{
//命名路由
name: 'xiangqing',
path: 'detile/:id/:title',
component: () => import('@/components/Detile.vue'),
// props的第一种写法,值为对象中的所有key-value都会以props的形式传递给组件
// props: { a: 1, b: 'hll' },
//props的第二种写法,值为布尔值。若布尔值为真,就会把该路由组件的所有params参数,以params的形式给组件
// props: true,
//props的第三种写法,值为函数
// props($route) {
// return { id: $route.params.id, title: $route.params.title }
// },
// 解构赋值
props({ params: { id, title } }) {
return { id, title }
},
},
router-link 的 replace 属性
- 作用:控制路由跳转时操作浏览器历史记录的模式
- 浏览器的来老司机了有两种写入方式:分别为 push 和 replace,push 时追加历史记录,replace 时替换当前记录。路由跳转时候默认时 push
- 如何开启 replace 模式:
<router-linkto= to="/about" :replace="true" class="list-group-item" active-class="active" >about</router-link >
编程时路由导航
- 作用:不借助
实现路由跳转,让路由跳转更灵活 - 具体编码
<button @click="pushShow(item)">push查看</button>
<button @click="replaceShow(item)">replace查看</button>
methods: {
pushShow(item) {
this.$router.push({
name: 'xiangqing',
params: {
id: item.id,
title: item.title,
},
})
},
replaceShow(item) {
this.$router.replace({
name: 'xiangqing',
params: {
id: item.id,
title: item.title,
},
})
},
},
-------------------------------------------------------------------------------
<button @click="back">后退</button>
<button @click="forward">前进</button>
<button @click="testGo">测试一下go</button>
methods: {
back() {
this.$router.back()
},
forward() {
this.$router.forward()
},
testGo() {
// 前进3位置 如果往后那就是负数
// this.$router.go(3)
this.$router.go(-3)
},
},
缓存路由组件
- 作用:让不展示的路由组件保持挂载,不被销毁
- 具体代码
<!-- 路由组件缓存 include="组件名" -->
<keep-alive include="neww">
<router-view></router-view>
</keep-alive>
不写include 那么都会缓存
有多的话 就这么写 :include="['组件名','组件名']"
两个新的钩子函数
- 作用:路由组件所独有的两个钩子,用于捕获路由组件的激活状态
- 具体名字:
- activated 路由组件被激活时触发
- deactivated 路由组件失效时触发
路由守卫
- 作用:对路由进行权限控制
- 分类:全局守卫,独享守卫,组件内守卫
全局守卫:
beforeEach(全局前置守卫)
// 全局前置路由守卫--初始化的时候被调用,每次路由切换之前被调用
router.beforeEach((to, from, next) => {
// 是否需要权限
if (to.meta.isAuth) { //判断当前路由是否需要进行权限控制
if (localStorage.getItem('name') === 'ab') { //权限控制的具体规则
next()
} else {
alert('你的名字不对')
}
} else {
next() //放行
}
})
afterEach(全局后置守卫)
// 全局后置路由守卫--初始化的时候被调用,每次路由切换之后被调用
router.afterEach((to, from) => {
//后置守卫这里配置title名称
if (to.meta.title) {
document.title = to.meta.title || 首页 //修改网页的title
} else {
document.title = 'packer.json文件里面的name'
}
})
独享路由守卫 beforeEnter
{
path: '/home',
name: 'zhuye',
meta: { title: '主页' },
component: () => import('@/components/home.vue'),
children: [
{
path: 'ness',
component: () => import('@/pages/neww.vue'),
meta: { isAuth: true, title: '下一个' }, //这个是路由元 谁需要权限的校验就选谁
// 独享路由守卫
beforeEnter: (to, from, next) => {
// 是否需要权限
if (to.meta.isAuth) { //判断当前路由是否需要进行权限控制
if (localStorage.getItem('name') === 'ab') { //权限控制的具体规则
next()
} else {
alert('你的名字不对')
}
} else {
next() //放行
}
}
组件内路由守卫
// 通过路由规则,进入该组件时被调用
beforeRouteEnter (to, from, next) {
// ...
},
// 通过路由规则,离开该组件时被调用
beforeRouteLeave (to, from, next) {
// ...
},
history 模式与 hash 模式
element-ui 的基本使用
element-ui 按需引入
小工具
nanoid: npm install –save nanoid
express: 搭建一个本地node服务器 npm i express
配置:
const express = require('express')
const app = express()
app.use(express.static(__dirname+'/static'))
app.get('/person', (req, res) => {
res.send({
name: 'tom',
age: 18,
})
})
app.listen(5050, (err) => {
if (!err) console.log('服务器启动成功了')
})
运行的话把静态资源放到static文件夹下面
运行:node server
connect-history-api-fallback: 用于解决history模式的404问题
在后端中下载 npm install –save connect-history-api-fallback
给文件中添加一句话
const history = require(‘connect-history-api-fallback’);
然后必须得在静态资源之前去使用
app.use(history())